Response compression refers to the process of compressing HTTP responses before sending them over the network.
This compression reduces the size of the response, which leads to faster transmission times and reduced bandwidth usage, leading to faster load times and improved performance, especially for web applications where bandwidth is a concern.
However, keep in mind that compression and decompression do add some overhead in terms of CPU usage.
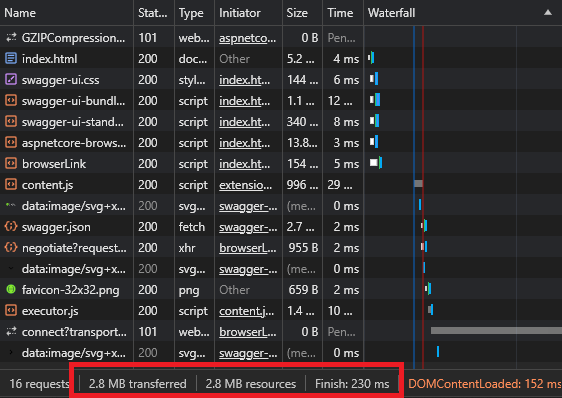
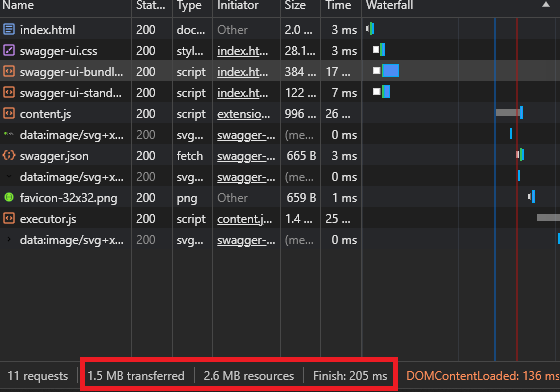
In .NET, you can enable response compression using middleware. Here’s how to do it:
Install the necessary packages
Ensure you have the Microsoft.AspNetCore.ResponseCompression
package installed.
You can do this via the NuGet Package Manager Console:
Install-Package Microsoft.AspNetCore.ResponseCompression
Configure response compression in your startup file
Open your Startup.cs
file and add the following code in the ConfigureServices
method:
using Microsoft.AspNetCore.Builder;
using Microsoft.Extensions.DependencyInjection;
using System.IO.Compression;
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddResponseCompression(options =>
{
options.EnableForHttps = true; // Enable compression for HTTPS responses
options.Providers.Add<GzipCompressionProvider>(); // Use Gzip compression
});
}
// Other configuration methods...
public void Configure(IApplicationBuilder app)
{
app.UseResponseCompression();
// Other middleware configuration
// app.UseRouting();
// app.UseEndpoints(endpoints => { endpoints.MapControllers(); });
}
}
In this code:
AddResponseCompression()
Method is used to add the response compression services to the service container.
UseResponseCompression()
is added to the request processing pipeline to enable compression for HTTP responses.
GzipCompressionProvider
is specified as the compression provider. You can also add other compression providers if needed.
EnableForHttps
option is set to True
it enables compression for HTTPS responses. This is optional, and you can choose to enable or disable it based on your requirements.
thanks for sharing
Hey people!!!!!
Good mood and good luck to everyone!!!!!